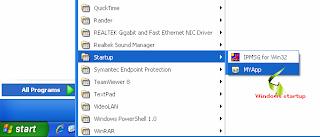
using IWshRuntimeLibrary;
using Microsoft.Win32;
using System.IO;
namespace Sample
{
public partial class Form1 : Form
{
RegistryKey reg = Registry.CurrentUser.OpenSubKey("SOFTWARE\\Microsoft\\Windows \\CurrentVersion\\Run", true); // check windows is run
private NotifyIcon tryIcon; //add icon in 'System Tray'
private ContextMenu trayMenu; // create menu
public Form1()
{
// if windows is run my app (setup1) is run
reg.SetValue("MyApp", Application.ExecutablePath);
MessageBox.Show("Test");
//create Menu Item
trayMenu = new ContextMenu ();
//Menu Item (Show, Hide, Exit) ==> menu Function (OnShow, OnHide, OnExit) in #region System Tray Menu
trayMenu.MenuItems.Add("Show", OnShow);
trayMenu.MenuItems.Add("Hide", OnHide);
trayMenu.MenuItems.Add("Exit", OnExit);
// Display icon in "System Tray" (refer image first)
tryIcon = new NotifyIcon(); // set Systen tray icon
tryIcon.Text = "Setup1"; // set system tray text
// how to add resources -> click 'Properties' -> open the 'Resources.resx' -> change to string to icon -> click 'Add Resource' - select 'Add Existing file' and select icon
tryIcon.Icon = Properties.Resources.ftprush_install; // add icon in 'System Tray'
tryIcon.ContextMenu = trayMenu; // add menu at "System try"
tryIcon.Visible = true; // Icon visible true
InitializeComponent();
}
void CreateStartupShortcut() // Display "Windows Startup" (refer image 2)
{
string startupFolder = Environment.GetFolderPath(Environment.SpecialFolder.Startup);
WshShell shell = new WshShell();
string shortcutAddress = startupFolder + @"\MYApp.lnk";
IWshShortcut shortcut = (IWshShortcut)shell.CreateShortcut(shortcutAddress);
shortcut.Description = "A startup shortcut. If you delete this shortcut from your computer, LaunchOnStartup.exe will not launch on Windows Startup"; // set the description of the shortcut
shortcut.WorkingDirectory = Application.StartupPath; /* working directory */
shortcut.TargetPath = Application.ExecutablePath; /* path of the executable */
// optionally, you can set the arguments in shortcut.Arguments
// For example, shortcut.Arguments = "/a 1";
shortcut.Save();
}
private void Form1_Load(object sender, EventArgs e)
{
this.Opacity = 0; // form not display (this is hide)
this.ShowInTaskbar = false; // display hide in Task bar
//this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.SizableToolWindow; // in Design mode:
}
#region System Tray Menu
private void OnExit(object sender, EventArgs e)
{
Application.Exit();
}
private void OnShow(object sender, EventArgs e)
{
this.Opacity =1; // form display (this is show)
this.ShowInTaskbar = true; // display in Task bar
}
private void OnHide(object sender, EventArgs e)
{
this.Opacity = 0; // form not display (this is hide)
this.ShowInTaskbar = false; // display hide in Task bar
}
#endregion System Tray Menu
}
}
Download Sample Coding (LaunchOnStartUp.rar)
Download Interop.IWshRuntimeLibrary.dll